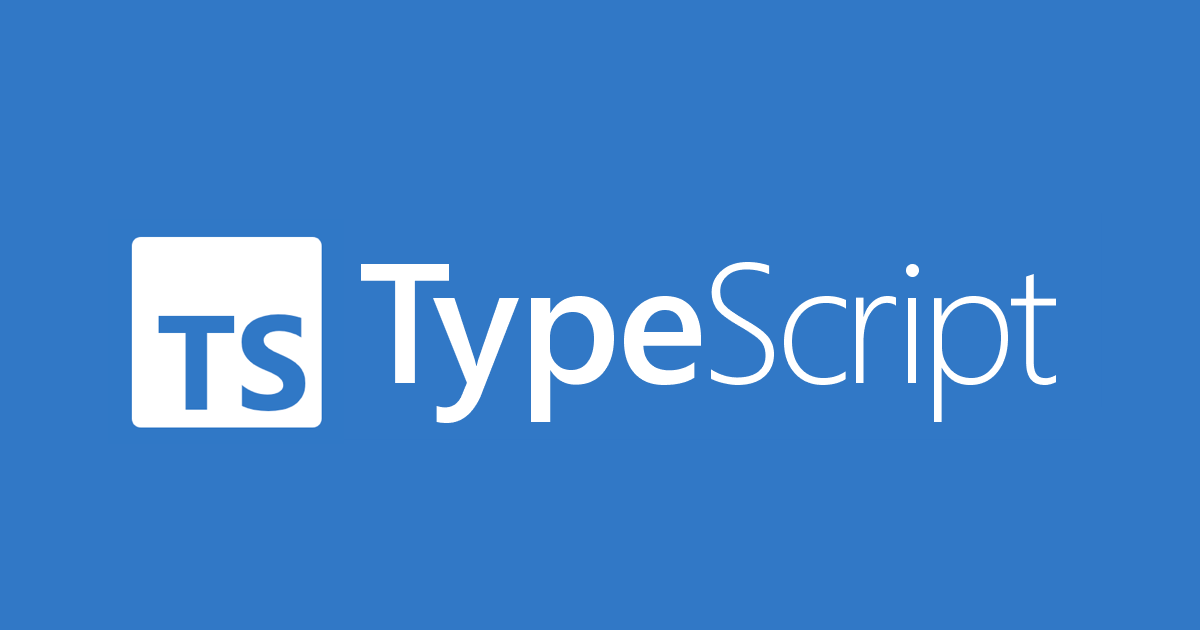

تایپ اسکریپت
21 مرداد 1402
تایپهای سودمند در تایپ اسکریپت
سلام دوستان امیدوارم خوب باشید و همیشه درحال یادگیری🧑💻. تایپهای سودمند یا Utility Type
ها تایپهای خاصی هستند که تایپاسکریپت در اختیار ما گذاشته تا تبدیل تایپ های رایج رو برامون انجام بده و کار برامون راحت تر بشه.
تایپ <Partial<Type
Partial
تمام پراپرتیهای یک تایپ را اختیاری میکنه. به مثال زیر توجه کنید:
interface Todo {
title: string;
description: string;
}
function updateTodo(todo: Todo, fieldsToUpdate: Partial<Todo>) {
return { ...todo, ...fieldsToUpdate };
}
در واقع Partial
مشابه کد زیر عمل میکنه:
interface Todo {
title?: string;
description?: string;
};
تایپ <Required<Type
تایپ Required
برخلاف Partial
عمل میکنه و تمام مقادیر را اجباری میکنه، حتی اگر پراپرتی optional
باشه.
interface Car {
make: string;
model: string;
mileage?: number;
}
let myCar: Required<Car> = {
make: 'Ford',
model: 'Focus',
mileage: 12000 // `Required` forces mileage to be defined
};
تایپ <Readonly<Type
Readonly
همانطور که از اسمش مشخص هست، یک اینترفیس یا تایپ را فقط خواندنی میکنه و نمیتوانیم هیچ تغییری ایجاد کنیم.
interface Todo {
title: string;
}
const todo: Readonly<Todo> = {
title: "Delete inactive users",
};
todo.title = "Hello"; // Cannot assign to 'title' because it is a read-only property.
اینو در نظر داشته باشید تایپاسکریپت زمان کامپایل جلوی این رو میگیره، اما از لحاظ تئوری چون به جاوا اسکریپت کامپایل میشه، در نتیجه هنوز هم میشه readonly
رو تغییر داد!
تایپ <Record<Keys, Type
Record
یک راه کوتاه هست برای تعریف تایپ آبجکت با دادن تایپ key
و تایپ value
آبجکت. Record
دو پارامتر دریافت میکنه که پارامتر اول، تایپ key
و پارامتر دوم، تایپ value
هستش.
interface CatInfo {
age: number;
breed: string;
}
type CatName = "miffy" | "boris" | "mordred";
const cats: Record<CatName, CatInfo> = {
miffy: { age: 10, breed: "Persian" },
boris: { age: 5, breed: "Maine Coon" },
mordred: { age: 16, breed: "British Shorthair" },
};
تایپ <Pick<Type, Keys
Pick
میاد تمام key
های یک آبجکت رو حذف میکنه بجز اون key
های که به پارامتر دوم Pick
میدیم، در نهایت تایپ جدید ایجاد میکنه.
interface Person {
name: string;
age: number;
location?: string;
}
const bob: Pick<Person, 'name'> = {
name: 'Bob'
// `Pick` has only kept name, so age and location were removed from the type and they can't be defined here
};
تایپ <Omit<Type, Keys
Omit
مشابه Pick
عمل میکند، یعنی یک تایپ جدید بر اساس تایپهای موجود ایجاد میکنه، با این تفاوت که پارامتر دوم، فیلدهایی هستند که میخواهیم در تایپ جدید وجود نداشته باشند.
interface Person {
name: string;
age: number;
location?: string;
}
const bob: Omit<Person, 'age' | 'location'> = {
name: 'Bob'
// `Omit` has removed age and location from the type and they can't be defined here
};
تایپ <Extract<Type, Union
Extract
به عنوان پارامتر اول تایپ مورد نظر را میگیره و به عنوان پارامتر دوم تایپی که میخوایم از تایپ اول استخراج کنیم را دریافت میکنه.
type Shape =
| { kind: "circle"; radius: number }
| { kind: "square"; x: number }
| { kind: "triangle"; x: number; y: number };
type T0 = Extract<Shape, { kind: "circle" }>
تایپ <NonNullable<Type
از NonNullable برای حذف مقادیر null و undefined استفاده میکنیم.
type NonnullableType = string | number | null | undefined;
type NoNulls = NonNullable<NonnullableType>;
تایپ <ReturnType<Type
استفاده از ReturnType
، تایپ خروجی تابع را مشخص میکنیم.
declare function f1(): { a: number; b: string };
type T0 = ReturnType<() => string>;
type T1 = ReturnType<(s: string) => void>;
type T2 = ReturnType<<T>() => T>;
type T3 = ReturnType<<T extends U, U extends number[]>() => T>;
type T4 = ReturnType<typeof f1>;
type T5 = ReturnType<any>;
type T6 = ReturnType<never>;
type T7 = ReturnType<string>;
// Type 'string' does not satisfy the constraint '(...args: any) => any'.
type T8 = ReturnType<Function>;
// Type 'Function' does not satisfy the constraint '(...args: any) => any'.
// Type 'Function' provides no match for the signature '(...args: any): any'.
خوب بچه ها امیدوارم این قسمت براتون مفید بوده باشه. خوشحال میشم اگه انتقادی یا پیشنهادی دارید برام بنویسید تا محتوای بهتری تولید بشه ❤️
منابع:
این پست برات مفید بود؟
0

0

0

0

نظرات